Static Properties & Methods in SystemVerilog: A Comprehensive Guide
Table of Contents
Introduction
In the world of ASIC Verification, understanding the intricacies of various programming constructs is paramount. Today, we’ll delve deep into the concept of STATIC properties and STATIC methods in SystemVerilog, their significance, and their practical applications.
1. When to Use STATIC Properties and STATIC Methods
STATIC properties are particularly useful when we need to share data across all instances of a class. For instance, we might use a STATIC property to keep track of the total number of instances of a class that have been created.
class MyClass;
static int instanceCount;
function new();
instanceCount++;
endfunction
endclass
In this example, instanceCount
is a STATIC property that increments every time a new instance of MyClass
is created.
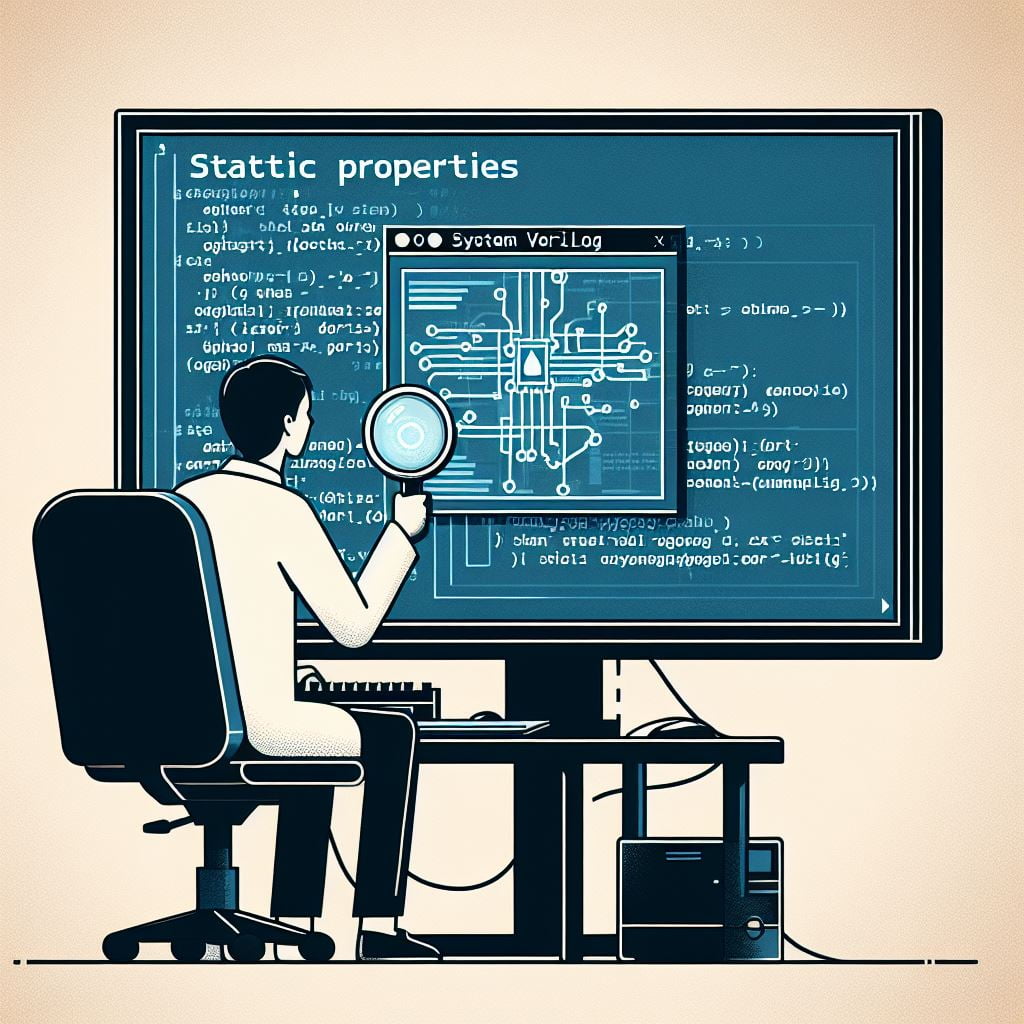
STATIC methods, on the other hand, are often used to create utility functions that are related to the class but don’t need to access any non-STATIC properties of the class.
class MyClass;
static function void printMessage();
$display("Hello, World!");
endfunction
endclass
In this example, printMessage()
is a STATIC method that prints a message to the console. It can be called without creating an instance of MyClass
.
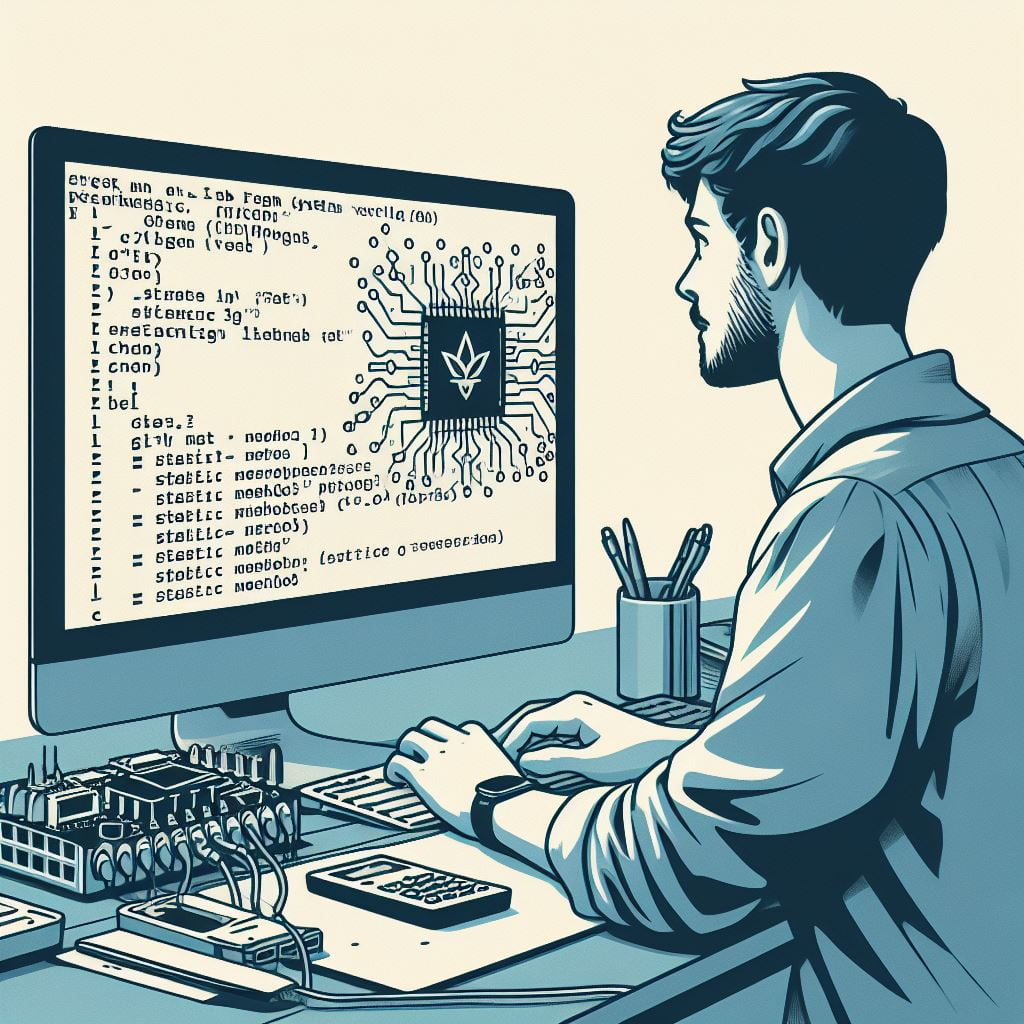
2. Practical Examples
Let’s consider a more complex scenario to illustrate the use of STATIC properties and methods. Suppose we have a class Car
with a STATIC property totalCars
and a method incrementTotalCars()
. Each time a new Car
is created, incrementTotalCars()
is called, updating totalCars
. This way totalCars
gives us the total number of Car
instances at any given time.
class Car;
static int totalCars; // STATIC property
// Constructor
function new();
incrementTotalCars(); // Call STATIC method
endfunction
// STATIC method
static function void incrementTotalCars();
totalCars++;
endfunction
// Method to get total cars
static function int getTotalCars();
return totalCars;
endfunction
endclass
module test;
initial begin
Car c1 = new();
Car c2 = new();
Car c3 = new();
$display("Total Cars: %0d", Car::getTotalCars()); // Access STATIC method
end
endmodule
In this example, we have a class Car
with a STATIC property totalCars
and two STATIC methods incrementTotalCars()
and getTotalCars()
. Every time a new Car
is created, incrementTotalCars()
is called, which increments totalCars
. We can then use the STATIC method getTotalCars()
to get the total number of Car
instances.
When you run this code, the output will be:
Total Cars: 3
This shows that three instances of the Car
class were created. The STATIC property totalCars
was shared across all instances of the class, allowing us to keep track of the total number of cars. This is a simple but powerful demonstration of how STATIC properties and methods can be used in SystemVerilog.
3. STATIC in a UVM Context
In a UVM environment, STATIC properties and methods can be leveraged in various ways. For example, a STATIC variable could be used in a UVM testbench to keep track of the total number of transactions across all sequences.
class MySequence extends uvm_sequence #(MyTransaction);
static int totalTransactions;
task body();
MyTransaction trans = MyTransaction::type_id::create("trans");
start_item(trans);
finish_item(trans);
totalTransactions++;
endtask
endclass
In this example, totalTransactions
is a STATIC property that increments every time a new transaction is started and finished in MySequence
.
4. Potential Pitfalls
While STATIC properties and methods can be very useful, they also come with potential pitfalls. One common issue is the risk of data being changed unexpectedly when shared across instances. It’s important to be aware of these issues and use STATIC properties and methods judiciously.
For example, if a STATIC property is used to store a value that should be unique for each instance of a class, unexpected behavior may occur because the value will be shared across all instances.
5. Conclusion
Understanding STATIC properties and methods in SystemVerilog is essential for effective ASIC Verification. By knowing when and how to use these constructs, we can write more efficient and maintainable code.
6. Case Studies
To provide a practical perspective, let’s look at some real-world case studies where STATIC properties and methods in SystemVerilog have been effectively used:
- Chip Verification: In a complex chip verification scenario, a STATIC property was used to keep track of the total number of verification cycles across all testbenches. This allowed the team to monitor the progress of the verification process in real time.
- Design Reuse: In a design reuse scenario, STATIC methods were used to create utility functions that could be used across multiple classes without the need for instantiation. This increased the efficiency and maintainability of the code.
7. Further Learning
To deepen your understanding of STATIC properties and methods in SystemVerilog, consider the following exercises:
- Create Your Examples: Try creating your classes with STATIC properties and methods. Experiment with different scenarios and see how STATIC properties and methods behave.
- Explore More Complex Scenarios: The examples provided in this guide are relatively simple. Try to come up with more complex scenarios where STATIC properties and methods could be useful.
- Read More: There are many great resources available online and in print that delve deeper into SystemVerilog and ASIC Verification. Some recommended books include “SystemVerilog for Verification” by Chris Spear and “Digital Design and Computer Architecture” by David Harris and Sarah Harris.
- Join a Community: There are many online communities where you can ask questions, share your knowledge, and learn from others. Consider joining forums or groups dedicated to SystemVerilog and ASIC Verification.
Remember, the best way to learn is by doing. Don’t be afraid to experiment and make mistakes. Happy learning!
static function int PKT_ID;
pkt_id++;
return pkt_id ;
endfunction: PKT_ID
endclass
There is a small mistake. The output of static function will be 1,2,3,4,5
Hi Hardik,
Firstly, Thank you for the blogs. Really nice.
I tried to run the 2nd chunk of code (static method). Saw the below output.
# dynapkt[0].id = 0
# dynapkt[1].id = 0
# dynapkt[2].id = 0
# dynapkt[3].id = 0
# dynapkt[4].id = 0
(Tool: Questa)
I am not able to ascertain the reason. Can you please explain that?